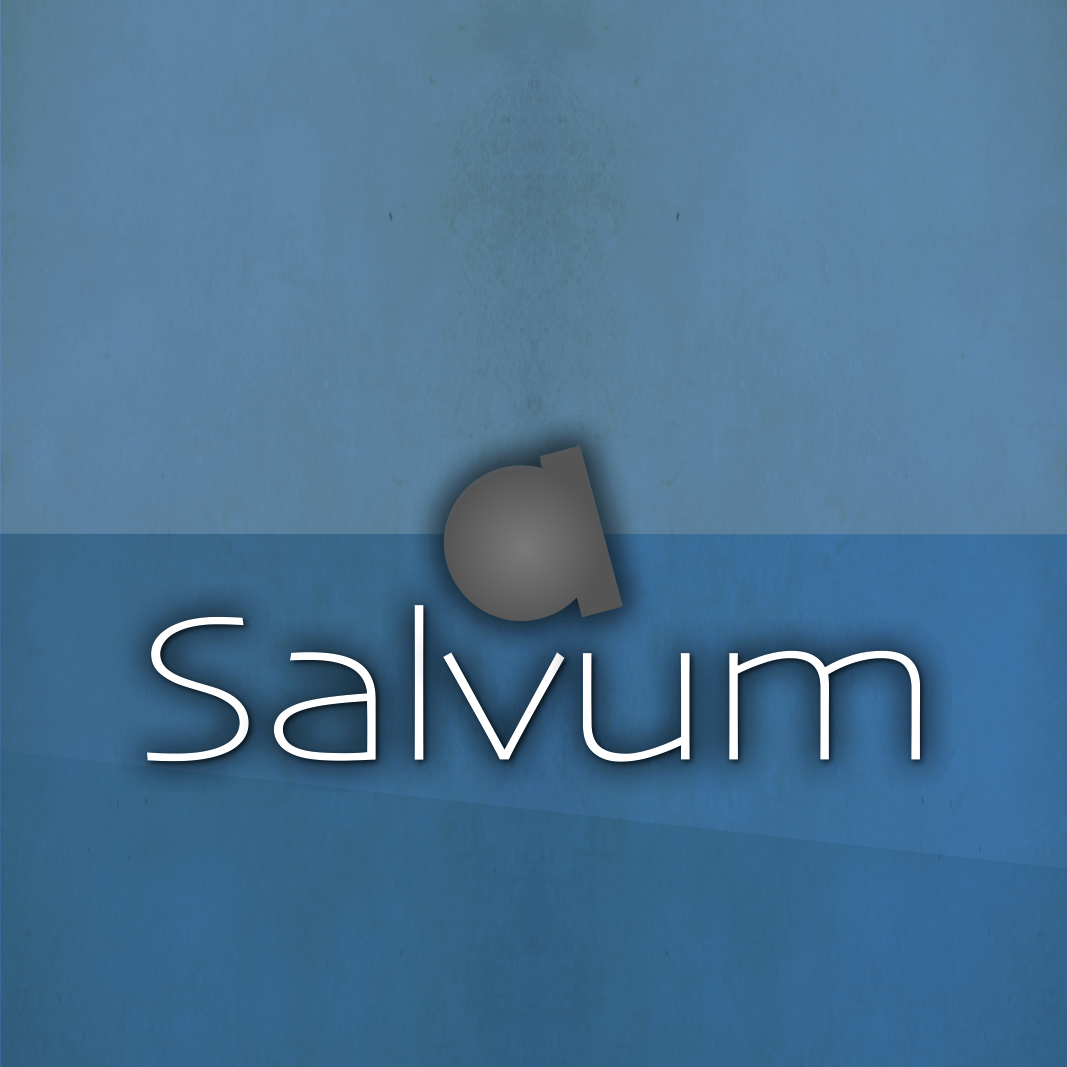
aSalvum 2.4
Organise your Pins and Authentications, and benefit from keep it always on your pocket
And now, on and for Apple Vision Pro
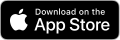
Small and very simple power manager schedule
Benefit from a small and very simple power manager schedule for macOs.
that will generate the necessary AppleScript inside your working directory. That you need execute after, so you can apply the pretended power schedule to your macOS
Totally developed using SwiftUI and Apple rock solid security principles.
These are some of the features that are ready to use.
Manage and schedule your macOS power on/off and more.
You have full control over what you run and when you run it.
With a user interface that is simple to understand and use.
Stay tuned because so much more is to come
Infinitum Authentication Salvum
These are some of the cool features.
So you can have access in all devices. *
To protect your sensitive information with Apple rock solid security principles.
With a user interface that is simple to understand and use.
Stay tuned because so much more is to come
* For use in multiple devices, you need to enable iCloud and also the iCloud KeyChain, so everything will be synched correctly between all the devices.
Organise your own inventory and benefit from keep it on your pocket.
An Home or Small Business Inventory platform, where you can organise your products by categories and Families.
You can benefit from permanent inventory in your pocket, where your can register all types of products (groceries, medication, furniture, electronic equipments...). Now, you only buy what you really need.
you can also provide to your insurance company a list of the items that you have at home.
And much more ...
These are some of the features that are ready to use.
You can export your products via email in csv format, for example to deliver to your insurance company.
You can organise your products by categories and Families.
You can add new products using the barcode reader.
With a user interface that is simple to understand and use.
A great network disk on your pocket.
These are some of the cool features.
You have access to your files using the embedded WebDAV Server.
You can share your files with other user using the shared folder.
You can import / export your files to iCloud.
With a user interface that is simple to understand and use.